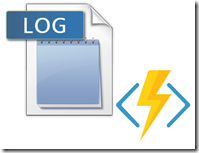
Logging is essential to the support of any piece of code. In this post I will cover two approaches to logging in Azure Functions: TraceWriter and log4net.
TraceWriter
The TraceWriter that is available out of the box with Azure Functions is a good starting point. Unfortunately it is short lived and only 1000 messages are kept at a maximum and at most they are held in file form for two days. That being said, I would not skip using the TraceWriter.
Your function will have a TraceWriter object passed to it in the parameters of the Run method. You can use the Debug, Error, Fatal, Info and Warn methods to write different types of messages to the log as shown below.
log.Info($"Queue item received: {myQueueItem}");
Once it is in the log you need to be able to find the messages. The easiest way to find the log files is through Kudu. You have to drill down from the LogFiles –> Application –> Functions –> Function –> <your_function_name>. At this location you will find a series of .log files if you function has been triggered recently.
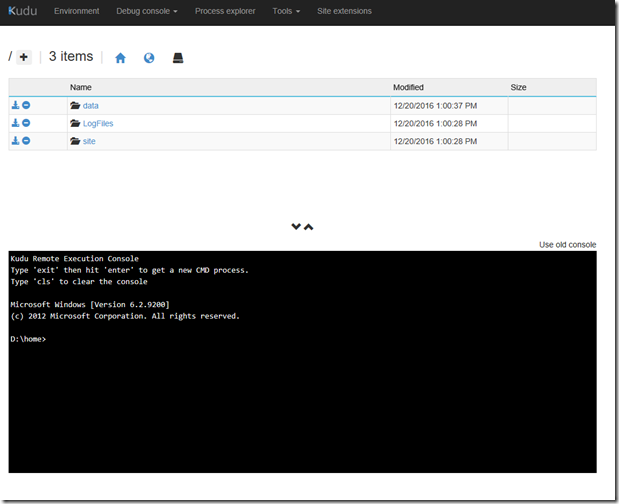
The other way to look at your logs is through Table Storage via the Microsoft Azure Storage Explorer. After attaching to your account open the storage account associated with your Function App. Depending on how you organized your resource groups you can find the storage account by looking at the list of resources in the group that the function belongs to.
Once you drill down to that account look for the tables named AzureWebJobHostLogsyyyymm as you see below.
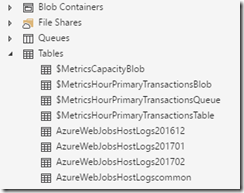
Opening these tables will allow you to see the different types of log entries saved by the TraceWriter. If you filter to the partition key “I” you will see the entries your functions posted. You can further filter name and date range to identify specific log entries.

log4net
If the default TraceWriter isn’t robust enough you can implement logging via a framework like log4net. Unfortunately because of the architecture of Azure Functions this isn’t as easy as it would be with a normal desktop or web application. The main stumbling block is the lack of ability to create custom configuration sections which these libraries rely on. In this section I’ll outline a process for getting log4net to work inside your function.
The first thing that we need is the log4net library. Add the log4net NuGet package by placing the following code in the project.json file.
{
"frameworks": {
"net46":{
"dependencies": {
"log4net": "2.0.5"
}
}
}
}
To get around the lack of custom configuration sections we will bind a blob file with your log4net configuration. Simply take the log4net section of and save it to a text file. Upload that to a storage container and bind it to your function using the full storage path.
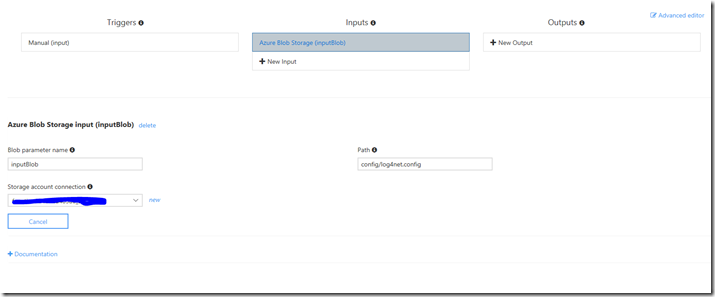
Add the references to the log4net library and configure the logger. Once you have that simply call the appropriate method on the logger and off you go. A basic sample of the code for configuring and using the logger is listed below. In this case I am actually using a SQL Server appender.
using System;
using System.Xml;
using log4net;
public static void Run(string input, TraceWriter log, string inputBlob)
{
log.Info($"Log4NetPoc manually triggered function called with input: {input}");
log.Info($"{inputBlob}");
XmlDocument doc = new XmlDocument();
doc.LoadXml(inputBlob);
XmlElement element = doc.DocumentElement;
log4net.Config.XmlConfigurator.Configure(element);
ILog logger = LogManager.GetLogger("AzureLogger");
logger.Debug($"Test log message from Azure Function", new Exception("This is a dummy exception"));
}
Summary
By no means does this post cover every aspect of these two logging approaches or all possible logging approaches for Azure Functions. In future posts I will also cover AppInsight. In any case it is always important to have logging for you application. Find the tool that works for your team and implement it.