In Worktile Pro we are using MongoDB and Mongoose as the backend database with Mongoose Node.js module for ODM (Object Document Mapping) framework.
Although Mongoose brings “Schema” back it still provides some advantages such as validation, abstraction, reference and default value. In this post I will talk about the default value based on a bug I found when developing Worktile Pro.
We can define default value against a model schema. In the code below I have a blog schema, with default value against “created_by” and “created_at” properties.
let BlogSchema = new Schema({
title : {type : String},
created_by : {type : String, default : 'Shaun Xu'},
created_at : {type : Number, default : Date.now}
});
If we tried to add a new blog document without specifying them, Mongoose will use default value as below.
let blog1 = new BlogModel({title : 'Implement Schema Inherence in Mongoose'});
co(function *() {
let savedBlog1 = yield blog1.save();
console.log(`Blog : $ { JSON.stringify(savedBlog1, null, 2) }`);
});
But if we specified “null”, they will be marked as “defined” so that Mongoose will set “null” rather than the default value.
let blog2 = new BlogModel({
title : 'Angular Toolkits: Customizable Bootstrap Tabs',
created_by : null,
created_at : null
});
co(function *() {
let savedBlog2 = yield blog2.save();
console.log(`Blog : $ { JSON.stringify(savedBlog2, null, 2) }`);
});
How about “undefined”? Let’s look at the code below. Will Mongoose use default value?
let blog3 = new BlogModel({
title : 'Pay Attention to "angular.merge" in 1.4 on Date Properties',
created_by : undefined,
created_at : undefined
});
The result was shown below, Mongoose didn’t use default value for “blog3”.
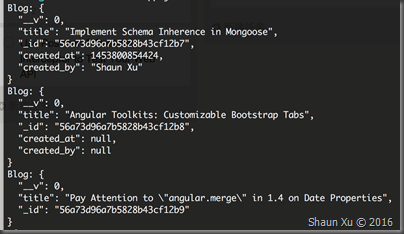
This means even though we set property to “undefined”, Mongoose will NOT use default value. This is because Mongoose just check if the document object contains properties. If yes, no matter it has value, it’s “null”, or even it’s “undefined”, it was defined. So Mongoose will NOT use default value.
So finally rule: If you want Mongoose to use default value on a property, do NOT specify it in the document object, even though set it to “null” or “undefined”.
Hope this helps,
Shaun